Bash Script: Perulangan while, until, for, select
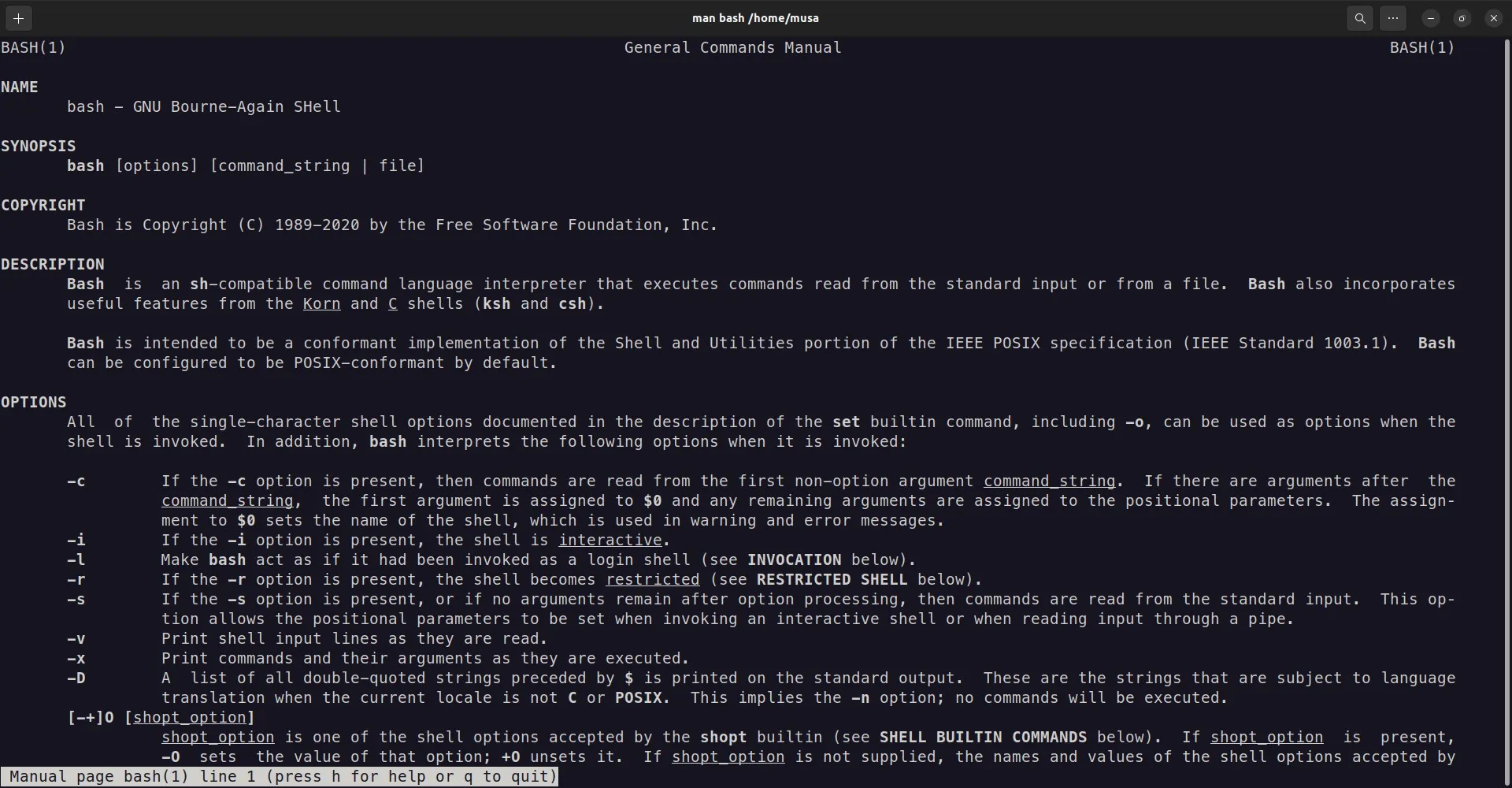
Artikel ini bagian dari Bash Script yang terdiri dari 7 bagian :
Perulangan menggunakan while
Format penulisan while
1 2 3 4 | while [ <some test> ] do <commands> done |
Contoh
1 2 3 4 5 6 7 8 9 | #!/bin/bash clear counter=1 while [ $counter -le 10 ] do echo $counter ((counter++)) done |
Hasilnya
1 2 3 4 5 6 7 8 9 10 | 1 2 3 4 5 6 7 8 9 10 |
Perulangan menggunakan until
Format penulisan until
1 2 3 4 | until [ <some test> ] do <commands> done |
Contoh
1 2 3 4 5 6 7 8 9 | #!/bin/bash clear counter=1 until [ $counter -gt 10 ] do echo $counter ((counter++)) done |
Hasilnya
1 2 3 4 5 6 7 8 9 10 | 1 2 3 4 5 6 7 8 9 10 |
Perulangan menggunakan for
Format penulisan for
1 2 3 4 | for var in <list> do <commands> done |
Contoh
1 2 3 4 5 6 7 8 9 | #!/bin/bash clear distros="Debian Ubuntu RedHat Slackware" for distro in $distros do echo $distro done |
Hasilnya
1 2 3 4 | Debian Ubuntu RedHat Slackware |
Contoh lain perulangan angka
1 2 3 4 5 6 7 | #!/bin/bash clear for angka in {1..10}; do echo "angka=$angka"; done |
Hasilnya
1 2 3 4 5 6 7 8 9 10 | angka=1 angka=2 angka=3 angka=4 angka=5 angka=6 angka=7 angka=8 angka=9 angka=10 |
Contoh lain perulangan angka dengan jarak lompatan 3
1 2 3 4 5 6 7 | #!/bin/bash clear for i in {0..13..3} do echo "$i" done |
Hasilnya
1 2 3 4 5 | 0 3 6 9 12 |
Perulangan menggunkan select
Dengan menggunakan select kita dapat membuat menu.
Format penulisan select
1 2 3 4 | select var in <list> do <commands> done |
Contoh
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | #!/bin/bash clear select DRINK in tea cofee water juice apple all none do case $DRINK in tea|cofee|water|all) echo "Go to canteen" ;; juice|apple) echo "Available at home" ;; none) break ;; *) echo "ERROR: Invalid selection" ;; esac done |
Hasilnya
1 2 3 4 5 6 7 8 9 10 | 1) tea 2) cofee 3) water 4) juice 5) apple 6) all 7) none #? 3 Go to canteen #? |
Contoh lain
1 2 3 4 5 6 7 8 9 10 11 12 13 | #!/bin/bash clear names='Goku Naruto Luffy Exit' echo 'Pilih karakter : ' select name in $names do if [ $name == 'Exit' ] then break fi echo "Hello $name" done |
Hasilnya
1 2 3 4 5 6 7 8 | Pilih karakter : 1) Goku 2) Naruto 3) Luffy 4) Exit #? 1 Hello Goku #? |
selamat mencoba 🙂
referensi:
pemula.linux.or.id
ryanstutorials.net