How to Deploy Go Web Application on Ubuntu
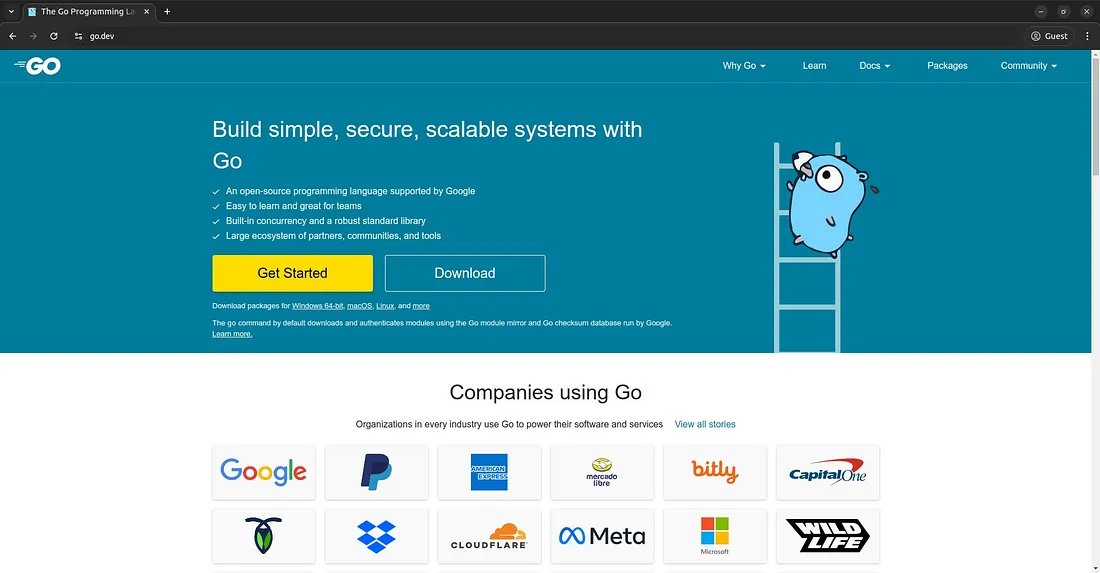
Go, also known as Golang, is an open-source programming language developed by Google. Go is designed for efficiency, ease of use, and scalability. It is very popular for building web applications, microservices, and cloud-based applications. In this guide, we will discuss how to deploy a simple Go web application on Ubuntu using Nginx as a reverse proxy and Systemd to manage the application service.
Install Go
Update and install Go:
1 2 3 4 5 | sudo apt update wget https://go.dev/dl/go1.23.3.linux-amd64.tar.gz sudo tar -C /usr/local -xzf go1.23.3.linux-amd64.tar.gz echo 'export PATH=$PATH:/usr/local/go/bin' >> ~/.profile source ~/.profile |
Verifying Go installation by displaying the version number:
1 | go version |
The response to the command:
1 | go version go1.23.3 linux/amd64 |
Creating a Go App
Create a directory for the application project with the name goweb:
1 | mkdir ~/goweb && cd ~/goweb |
Creating the main.go file:
1 | nano main.go |
The contents of the file main.go:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | package main import ( "fmt" "net/http" ) func handler(w http.ResponseWriter, r *http.Request) { fmt.Fprintf(w, "Hello, World!") } func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil) } |
Compile the application:
1 | go build -i app main.go |
Creating a Service
Create a systemd service file:
1 | sudo nano /etc/systemd/system/goweb.service |
File contents:
1 2 3 4 5 6 7 8 9 10 11 12 13 | [Unit] Description=Go Web App After=network.target [Service] ExecStart=/home/youruser/goweb/app Restart=always User=youruser Group=youruser Environment=PORT=8080 [Install] WantedBy=multi-user.target |
Enable the service:
1 2 3 4 | sudo systemctl daemon-reload sudo systemctl enable goweb sudo systemctl start goweb sudo systemctl status goweb |
Nginx Configuration
Install Nginx:
1 | sudo apt install nginx -y |
Create the Nginx configuration file:
1 | sudo nano /etc/nginx/conf.d/goweb.conf |
File contents:
1 2 3 4 5 6 7 8 9 10 11 12 13 | server { listen 80; server_name yourdomain.com; location / { proxy_pass http://localhost:8080; proxy_http_version 1.1; proxy_set_header Upgrade $http_upgrade; proxy_set_header Connection 'upgrade'; proxy_set_header Host $host; proxy_cache_bypass $http_upgrade; } } |
Restart Nginx:
1 | sudo systemctl restart nginx |
Test the Application
Open your browser and visit the address:
1 | http://yourdomain.com |
The result is the text “Hello, World!”.