How to Deploy Rust Web Application on Ubuntu
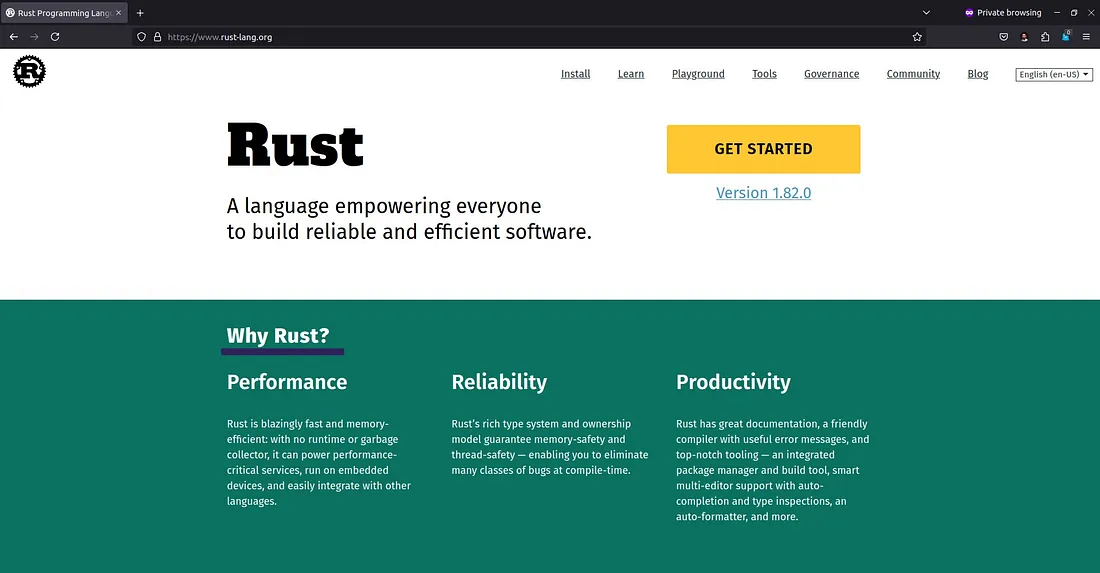
Rust is a programming language known for its high performance, memory safety without a garbage collector, and its ability to build fast and efficient applications. One of the reasons Rust is becoming increasingly popular is its ease of use for building web applications with various frameworks available, such as Actix Web and Rocket.
Rust also has Cargo, a great build and package manager that makes managing dependencies and compiling applications easy. By using Crates.io, Rust has a vast ecosystem of libraries (crates), allowing us to build complex applications with minimal code.
In this tutorial, we will create a simple web application, compile it, and then deploy it using Nginx as a reverse proxy.
Install Dependencies and Rust
Update and install dependencies:
1 2 | sudo apt update sudo apt install build-essential -y |
Install Rust:
1 | curl https://sh.rustup.rs -sSf | sh |
Verify Rust and Cargo installation:
1 2 3 | rustup --version rustc --version cargo --version |
The resulting response:
1 2 | rustc 1.82.0 (f6e511eec 2024-10-15) cargo 1.82.0 (8f40fc59f 2024-08-21) |
Membuat Rust App
Create a directory for the Rust project:
1 2 | cargo new rustweb cd rustweb |
Opening the src/main.rs file:
1 | nano src/main.rs |
Replace the contents with the following code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | use std::io::prelude::*; use std::net::{TcpListener, TcpStream}; fn handle_connection(mut stream: TcpStream) { let mut buffer = [0; 512]; stream.read(&mut buffer).unwrap(); let response = "HTTP/1.1 200 OK\r\nContent-Type: text/html; charset=UTF-8\r\n\r\n <html><body>Hello, World!</body></html>"; stream.write(response.as_bytes()).unwrap(); stream.flush().unwrap(); } fn main() { // Bind server to localhost:8000 let listener = TcpListener::bind("127.0.0.1:8000").unwrap(); println!("Server running on http://127.0.0.1:8000"); for stream in listener.incoming() { let stream = stream.unwrap(); handle_connection(stream); } } |
Compile the application:
1 | cargo build --release |
The application’s binary will be located in target/release/rustweb.
Creating a Service
Creating a systemd service file:
1 | sudo nano /etc/systemd/system/rustweb.service |
File contents:
1 2 3 4 5 6 7 8 9 10 11 12 13 | [Unit] Description=Rust Web App After=network.target [Service] User=youruser Group=youruser WorkingDirectory=/home/youruser/rustweb ExecStart=/home/youruser/rustweb/target/release/rustweb Restart=always [Install] WantedBy=multi-user.target |
Enable the service:
1 2 3 4 | sudo systemctl daemon-reload sudo systemctl enable rustweb sudo systemctl start rustweb sudo systemctl status rustweb |
Nginx Configuration
Install Nginx:
1 | sudo apt install nginx -y |
Create the Nginx configuration file:
1 | sudo nano /etc/nginx/conf.d/rustweb.conf |
File contents:
1 2 3 4 5 6 7 8 9 10 11 | server { listen 80; server_name example.com; location / { proxy_pass http://127.0.0.1:8000; proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; } } |
Restart Nginx:
1 | sudo systemctl restart nginx |
Test the Application
Open your browser and visit the address:
1 | http://example.com |
The result is the text “Hello, World!”.